All AP Computer Science A Resources
Example Questions
Example Question #25 : Program Implementation
CONSTRUCTORS
Consider the following Java code:
class Person
{
String firstName;
String lastName;
int age;
Person(String l, String f, int a)
{
firstName = f;
lastName = l;
age = a;
}
}
In main, how can I correctly create a person who is 21 years old, whose first name is Indiana, and whose last name is Jones?
Person person1 = new Person();
Person person1 = new Person("Jones", "Indiana", 21);
Person person1 = new Person("Indiana", "Jones", 21);
Person person1 = new Person("Jones", "Indiana", "21");
Person person1 = new Person("Jones", "Indiana", 21);
When we take a look at the constructor, first we notice that it has three parameters. Since there isn't any constructor overloading, this means that we can only create a person if we provide all of the arguments. So we can eliminate Person person1 = new Person() because that answer is not providing any arguments.
Now, we are supposed to be providing first two String arguments and then an integer. Therefore, we can eliminate the following two answers:
Person person1 = new Person("Jones", "Indiana", "21");
Person person1 = new Person(21, "Indiana", "Jones");
We can eliminate the first because the number 21 is being passed as a String instead of an int. It's being passed as a String because it has the double quotation. We can also eliminate the second because an integer is being passed as the first argument, instead of the third.
Lastly whenever a new object is created, the order of the arguments matter! Notice that the constructor is assigning the first parameter "l" to lastName. Therefore, our first argument should be "Jones". Next, the constructor takes the second parameter "f" and assigns it to the variable firstName. This means that our second argument should be "Indiana". Lastly, the constructor takes the third parameter "a" and assigns it to the integer variable age. This means that the last argument we should pass is the number 21 (without any quotes). This means that the correct answer is:
Person person1 = new Person("Jones", "Indiana", 21);
Example Question #31 : Programming Constructs
Create the method stub for a void method called ingredients that takes in spaghetti, sauce, and meatballs as parameters where the parameters are all strings.
ingredients(spaghetti, sauce, meatballs);
void ingredients(String spaghetti, String sauce, String meatballs);
void ingredients(spaghetti, sauce, meatballs);
ingredients(String spaghetti, String sauce, String meatballs);
void ingredients(String spaghetti, String sauce, String meatballs);
The answer choice is correct because it defines a void method (void ingredients) that takes in the three string parameters (String spaghetti, String sauce, String meatballs). The other answer choices are missing either the void type on the method stub or the type of parameter. Note both the return type of the method (void in this example) and the types of the parameters are very important.
Certified Tutor
All AP Computer Science A Resources
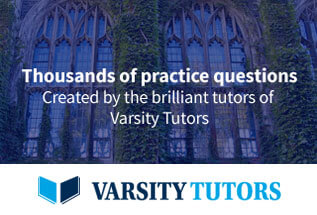