All AP Computer Science A Resources
Example Questions
Example Question #1 : Program Design
Consider the code below:
private static class Philosopher {
private String name;
private String favoriteSubject;
public Philosopher(String n, String f) {
name = n;
favoriteSubject = f;
}
public String getName() {
return name;
}
public String getFavoriteSubject() {
return favoriteSubject;
}
public void speak() {
System.out.println("Hello, World! My name is "+name + ". My favorite subject is "+favoriteSubject);
}
}
private static class Nominalist extends Philosopher {
boolean franciscan;
public Nominalist(String n,boolean frank) {
super(n,"logic");
franciscan = frank;
}
public void speak() {
super.speak();
if(franciscan) {
System.out.println("I am a Franciscan");
} else {
System.out.println("I am not a Franciscan");
}
}
public String whoMightHaveTaughtMe() {
if(franciscan) {
return "Perhaps William of Ockham?....";
} else {
return "Perhaps it was Durandus of St. Pourçain — scandalous, a Dominican nominalist!";
}
}
}
If you wished to make a toString() method for both Philosopher and Nominalist, using the same output as in each class' current speak() methods, what would be the best way to organize your code?
I. Have toString() invoke both classes' speak() methods.
II. Have the speak() method invoke the new toString() method.
III. Create a new subclass and write the toString() method in that.
I
I and III
III
II
II
The toString() method must return a string value. Therefore, it makes the most sense that you would have this method generate the output that you currently generate in speak(). Then, you could have speak() invoke the toString() method to output the same string data.
Certified Tutor
All AP Computer Science A Resources
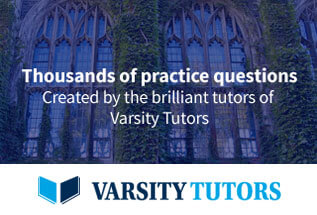