All AP Computer Science A Resources
Example Questions
Example Question #4 : Object Oriented Program Design
USING STATIC VARIABLES
CONSIDER THE FOLLOWING JAVA CODE:
public class Employee
{
static int nextID = 1;
String name;
int employeeID;
Employee(String n){
name = n;
employeeID = nextID;
nextID++;
}
public String getName(){
return name;
}
public int getID(){
return employeeID;
}
public static void main(String[] args)
{
Employee emp3 = new Employee("Kate");
Employee emp1 = new Employee("John");
Employee emp2 = new Employee("Sandy");
System.out.println(emp1.getName() + " ID: " + emp1.getID());
System.out.println(emp3.getName() + " ID: " + emp3.getID());
}
}
WHAT WOULD BE THE CONSOLE OUTPUT?
John ID: 2
Kate ID: 1
John ID: 1
Sandy ID: 2
John ID: 1
Sandy ID: 1
John ID: 1
Kate ID: 2
John ID: 2
Kate ID: 1
Static variables are associated with a class, not objects of that particular class. Since we are using a static class variable "nextID" and assigning that to employeeID as a new employee is created and then increasing it, the class variable is updated for the whole class.
In our code, we have emp3 being created first. This means that Kate is created with an employeeID equaling the current value of nextID (which is 1 in this case). Afterwards, the nextID value increases by 1. Next emp1 is created with the name of John and an employeeID equaling to the current value of nextID (which became 2 due to the increased that occurred when Kate was created). Lastly, emp2 is created with the name of Sandy and an employeeID equaling the current value (at that point in the code) of nextID. Meaning Sandy has an employeID value of 3.
Therefore in the first System.out. print statement when we print emp1, we get: John ID: 2
and in the second System.out. print statement when we print emp3 we get:
Kate ID: 1
Example Question #5 : Object Oriented Program Design
What is the relationship between the class Car and the class TwoDoor in the code snippet below?
class TwoDoor extends Car {
}
TwoDoor is the super class to Car
Car is the super class to TwoDoor
TwoDoor implements Car
Car implements TwoDoor
Car is the super class to TwoDoor
Car is the super class to TwoDoor. Since the class TwoDoor extends the class Car, TwoDoor can use methods and variables from Car by calling super.
The answers that mention implements are incorrect because implementing refers to interfaces. For TwoDoor to implement car, the code would look like this:
class TwoDoor implements Car {
}
All AP Computer Science A Resources
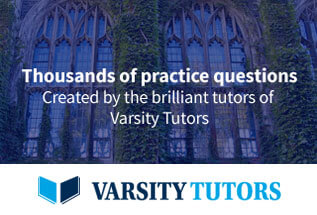