All AP Computer Science A Resources
Example Questions
Example Question #41 : Program Analysis
Which of the following code excerpts would output "10"?
None of the answers are correct.
int num = 5;
num = (num < 0) ? 10: 11;
System.out.println(num);
int num = 5;
num = (num > 0) ? 11: 10;
System.out.println(num);
int num = 5;
num = (num > 0) ? 10: 11;
System.out.println(num);
int num = 10;
num = (num > 0) ? 11: 12;
System.out.println(num);
int num = 5;
num = (num > 0) ? 10: 11;
System.out.println(num);
Each bit of code has something similar to this:
num = (boolean statement) ? X : Y;
The bit at the end with the ? and : is called a ternary operator. A ternary operator is a way of condensing an if-else statement. A ternary operator works like this:
<boolean statement> ? <do this if true> : <do this if false>
The correct answer is
int num = 5;
num = (num > 0) ? 10: 11;
System.out.println(num);
Therefore, the ternary operator portion of code, when converted to an if-else, looks like this:
if (num > 0) {
num = 10;
else {
num = 11;
}
num
is 5, which is greater than 0, it would go into the if
, so num
would then get 10. Then, num
gets printed, which means 10 gets printed (the correct answer).Example Question #2 : Program Correctness
True or False.
The assertion in this code snippet is to prevent users from inputting bad data.
public class UserInput {
int userInput;
public static void main(String[] args) {
assertTrue(isInteger(args[0]));
userInput = args[0];
userInput = 25 - userInput;
}
}
True
False
True
The assertion is used to validate user input. The user is able to input anything into the value at args[0]. Since this is the case, we must validate and make sure that the user is inputting what we want. To make this code snippet better, add error checking to do something if the user did not input an integer.
Certified Tutor
All AP Computer Science A Resources
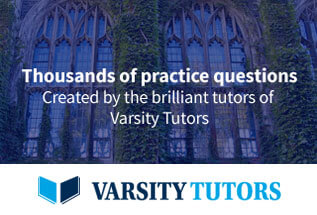