All AP Computer Science A Resources
Example Questions
Example Question #1 : Implementation Techniques
Consider the following code:
public static class Clock {
private int seconds;
public Clock(int s) {
seconds = s;
}
public void setTime(int s) {
seconds = s;
}
public void setSeconds(int s) {
int hoursMinutes = seconds - seconds % 60;
seconds = hoursMinutes + s;
}
public void setMinutes(int min) {
int hours = seconds / 3600;
int currentSeconds = seconds % 60;
seconds = hours + min * 60 + currentSeconds;
}
}
Which of the following represents a method that returns the minute value of the clock?
public int getMinutes() {
return seconds / 60;
}
public int getMinutes() {
return seconds * 60;
}
public int getMinutes() {
int hours = seconds / 3600;
return (seconds - hours * 3600);
}
public int getMinutes() {
return mins;
}
public int getMinutes() {
int hours = seconds / 3600;
return (seconds - hours * 3600) / 60;
}
public int getMinutes() {
int hours = seconds / 3600;
return (seconds - hours * 3600) / 60;
}
This clock class is defined as having only seconds in its fields, so you have to convert this value for any of the accessors and mutators. Therefore, you need to perform careful (though simple) mathematics for this conversion. To calculate the number of minutes in a given number of seconds, you first need to remove the hours from total count. First, compute the hours using integer division (which will drop the fractional portion of the decimal). This is:
int hours = seconds / 3600;
Next, you need to figure out how many seconds are in that value of hours. This is helpful precisely because integer division drops the decimal portion. Thus, you will subtract off:
hours * 3600
from the total seconds.
This gives you the seconds that apply to the minutes and seconds of the time. Therefore, you will finally need to divide by 60 to isolate the minutes.
Example Question #1 : Information Hiding
Consider the following code:
public class Clock {
private int seconds;
public Clock(int s) {
seconds = s;
}
public void setTime(int s) {
seconds = s;
}
public void setSeconds(int s) {
int hoursMinutes = seconds - seconds % 60;
seconds = hoursMinutes + s;
}
public void setMinutes(int min) {
int hours = seconds / 3600;
int currentSeconds = seconds % 60;
seconds = hours + min * 60 + currentSeconds;
}
}
Which of the following defines a toString method that will output the time in 24-hour format in the following form:
1:51:03
(Notice that you need to pad the minutes and seconds. You can call 12 midnight "0".)
public String toString() {
int hours = seconds / 3600;
int mins = (seconds - hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString += "0";
}
int secs = seconds % 60;
String secString = "" + secs;
if(secs < 10) {
secString += "0";
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 60;
int mins = seconds - (hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds * 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 3600;
int mins = seconds - (hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds * 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 3600;
int mins = (seconds - hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds % 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 3600;
int mins = (seconds - hours * 3600);
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds % 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
public String toString() {
int hours = seconds / 3600;
int mins = (seconds - hours * 3600) / 60;
String minString = ""+mins;
if(mins < 10) {
minString = "0" + minString;
}
int secs = seconds % 60;
String secString = "" + secs;
if(secs < 10) {
secString = "0" + secString;
}
return hours + ":"+minString+":"+secString;
}
There are several points to be noted in this code. First, you need to calculate each of the constituent parts from the seconds stored in the class. The "display value" of seconds is relatively easy. This is just the remainder of a division by 60. Consider:
61 seconds => This is really 1 minute and 1 second.
155 seconds => This is really 2 minutes and 35 seconds.
So, you know that the display seconds are:
seconds % 60
You must compute the hours using integer division (which will drop the fractional portion of the decimal). This is:
int hours = seconds / 3600;
Next, you need to figure out how many seconds are in that value of hours. This is helpful precisely because integer division drops the decimal portion. Thus, you will subtract off:
hours * 3600
from the total seconds.
This gives you the seconds that apply to the minutes and seconds of the time. Therefore, you will finally need to divide by 60 to isolate the minutes.
Then, you must pad the values. This is not done using +=
, which would add the "0" character to the end of the string. Instead, it is done by the form:
secString = "0" + secString;
All AP Computer Science A Resources
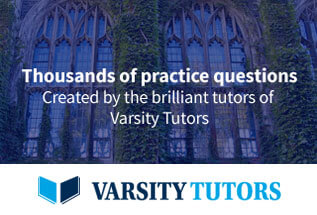