All AP Computer Science A Resources
Example Questions
Example Question #1 : Identifying & Correcting Errors
Consider the following code:
int num = 1;
for(int i = 0; i < 10; i++) {
num *= i;
}
The code above is intended to provide the continuous product from num to 10. (That is, it provides the factorial value of 10!.) What is the error in the code?
You cannot use *= in a loop.
The loop control is incorrect.
The i initialization is wrong.
Both the i intialization and the loop control value are incorrect.
The loop control, the i initialization, and the initialization for num are all wrong.
Both the i intialization and the loop control value are incorrect.
This loop will indeed run 10 times. However, notice that it begins on 0 and ends on 10. Thus, it will begin by executing:
num *= 0
Which is the same as:
num = num * 0 = 0
Thus, you need to start on 1 for i. However, notice also that you need to go from 1 to 10 inclusive. Therefore, you need to change i < 10 to i <= 10.
Example Question #1 : Identifying & Correcting Errors
Consider the code below:
public class Clock {
private int seconds;
public Clock(int s) {
seconds = s;
}
public void setTime(int s) {
seconds = s;
}
public void setSeconds(int s) {
int hoursMinutes = seconds - seconds % 60;
seconds = hoursMinutes + s;
}
public void setMinutes(int min) {
int hours = seconds / 3600;
int currentSeconds = seconds % 60;
seconds = hours + min * 60 + currentSeconds;
}
}
Which of the following issues could be raised regarding the code?
There is a potential null pointer exception in one of the methods.
The setMinutes
method should return an integer value.
There is insufficient data verification for the mutator methods.
There are no issues.
There is an error in the setMinutes
calculation.
There is insufficient data verification for the mutator methods.
Recall that a "mutator" is a method that allows you to change the value of fields in a class. Now, for the setTime
, setMinutes
, and setSeconds
methods, you do not check several cases of errors. For instance, you could give negative values to all of these methods without causing any errors to be noted. Likewise, you could assign a minute or second value that is greater than 60, which could cause errors as well. Finally, you could even make the clock have a value that is greater than "23:59:59", which does not make much sense at all!
Example Question #1 : Identifying & Correcting Errors
What is wrong with the following code?
- int main()
- {
- bool logic;
- double sum=0;
- for(j=0;j<3;j++)
- {
- sum=sum+j;
- }
- if (sum>10)
- {
- logic=1;
- }
- else
- {
- logic=0;
- }
- }
- }
There is an opening/closing bracket missing.
You cannot define the variable logic as a bool.
The code has no errors.
You must define the sum variable as an integer, not a double.
Variable j is not defined.
Variable j is not defined.
If you notice, in our for loop, the integer j is used as the iteration variable. However, no where in the code is that variable defined. To fix this issue this could have been done.
for (int j=0;j<3,j++)
Note the bold here is inserted. We need to define j here. We could have also defined j as a double.
Example Question #3 : Identifying & Correcting Errors
FILE INPUT/OUTPUT
Consider the following C++ code:
1. #include <iostream>
2. #include <fstream>
3. using namespace std;
4. int main() {
5. ifstream outputFile;
6. inputFile.open("TestFile.txt");
7. outputFile << "I am writing to a file right now." << endl;
8. outputFile.close();
9. //outputFile << "I'm writting on the file again" << endl;
10. return 0;
11. }
What is wrong with the code?
Testfile.txt shouldn't have double quotations around it.
The ifstream library should be included.
There is nothing wrong with the code.
The outputFile should be of type "ofstream", not "ifstream".
The outputFile should be of type "ofstream", not "ifstream".
Type ifstream objects are used to read from files, NOT to write to a file. To write to a file you can use ofstream. You can also you fstream to both read and write to and from a file.
Example Question #252 : Computer Science
a. public void draw() {
b. int i = 0;
c. while(i < 15){
d. system.out.println(i);
e. i++
f. }
g. }
Which lines of code have errors?
d e
e
b
cb
d c
d e
line d's error is that system.out.println (i); is not capitalized.
line e's error is that there is a missing semicolon.
All AP Computer Science A Resources
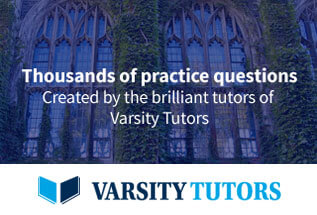