All AP Computer Science A Resources
Example Questions
Example Question #11 : Debugging
Consider the following code:
public static int[] del(int[] a,int delIndex) {
if(delIndex < 0 || delIndex >= a.length) {
return null;
}
int[] ret = new int[a.length - 1];
for(int i = 0; i < a.length; i++) {
if(i != delIndex) {
ret[i] = a[i];
}
}
return ret;
}
What is the error in the code above?
The loop is infinite.
You need to implement a swap for the values.
The intial conditional (i.e. the if statement) has incorrect logic.
The use of the array index i is incorrect.
There is a null pointer exception.
The use of the array index i is incorrect.
The problematic line in the code above is the one that reads:
ret[i] = a[i];
This is going to work well until the end of the array. The variable i is going to go for the length of the array a. However, the array ret is one less in length. This means that you will overrun your array, getting an ArrayIndexOutOfBoundsException at the very end of the looping. You would need to implement two indices—one of which will not be incremented on that one time when you skip the element that is removed from the array.
Example Question #13 : Program Analysis
What is wrong with this loop?
int i = 0;
int count = 20;
int[] arr = [1, 3, 4, 6, 8, 768, 78, 9]
while (i < count) {
if (i > 0) {
arr[i] = count;
}
if (i < 15) {
System.out.println("HEY");
}
}
if statement is incorrect
it prints Hey too many times
The loop is infinite
count never gets decremented
The loop is infinite
The loop is infinite because i never gets incremented to become greater than count.
The loop in this case is,
while (i < count) {
if (i > 0) {
arr[i] = count;
}
and the error occurs because there is no terminating factor in the while loop. A terminating factor would be,
while(i<count){
if(i>0, i<10, i++){
arr[i] = count;
}
Certified Tutor
All AP Computer Science A Resources
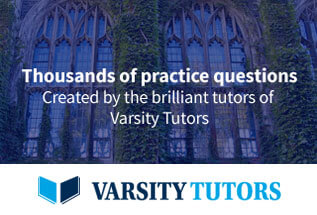