All Computer Science Resources
Example Questions
Example Question #2 : Program Design
Given a class Thing and the code:
Thing thingOne, thingTwo;
What function call is the following equivalent to?
thingTwo = thingOne;
None of the above
thingTwo.operator = (thingOne);
thingOne uses the copy constructor of thingTwo
ostream& Thing::operator=(const Thing& rhs);
operator=(thingTwo, thingOne);
thingTwo.operator = (thingOne);
What's given to us is that thingOne and thingTwo have already been created, this is a vital piece of information. Let's go through each of the choices
operator=(thingTwo, thingOne);
This line of code makes no sense and is syntactically wrong.
ostream& Thing::operator=(const Thing& rhs);
This line of code says to access the "operator=" method from the "Thing" class and put it onto the ostream, this has nothing to do with assigning thingOne to thingTwo.
When we come across the choice of the copy constructor, we have to keep in mind that the copy constructor is only used when an object use to to create another one, for example we have a given class called Foo:
Foo foo;
Foo foobar = foo;
In this case, we are using foo to create foobar.
The only choice that works is:
thingTwo.operator=(thingOne);
This line of code means that the thingOne object is being assigned to thingTwo.
Certified Tutor
All Computer Science Resources
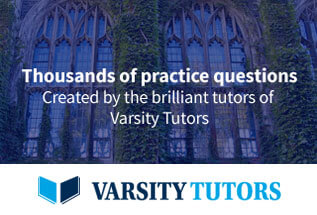